What are Django models?
A Django model is a built-in feature that is used to create tables and fields. These tables and fields are used to represent data that can be managed and accessed with Django. Simply put, models define the structure of the data that is stored.
Let's get started.
So, what we want to understand is how to read records from our table of cars based on our model Car. We will first look at how we can read all the records and then how to read a single record.
1) Reading all the records
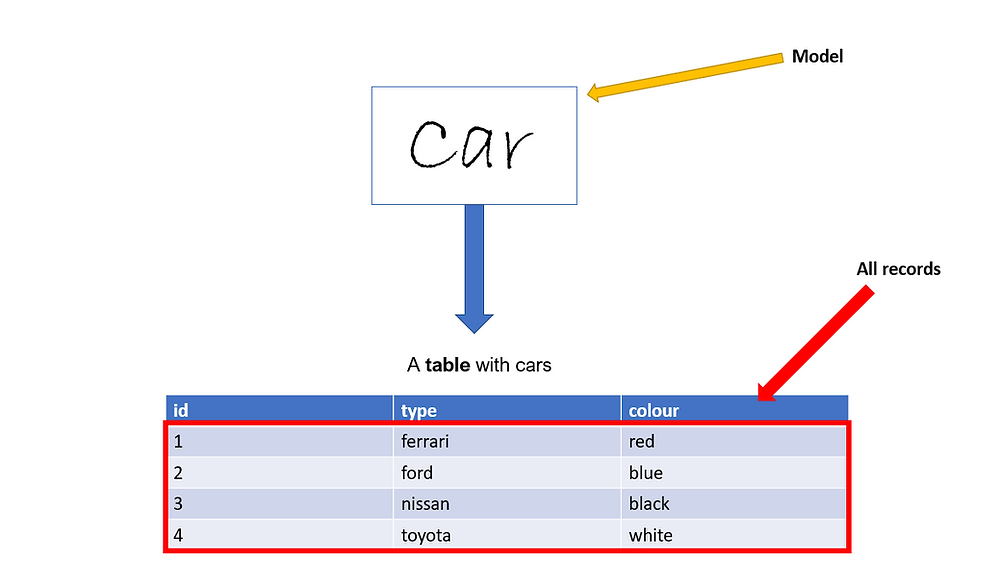
To be able to select and read all the records you need to invoke the all() function, which will give you access to all the cars (records) in the cars table.
The code below shows how we would read all the records.
# - views.py
cars = Car.objects.all()
2) Reading a single record (instance)
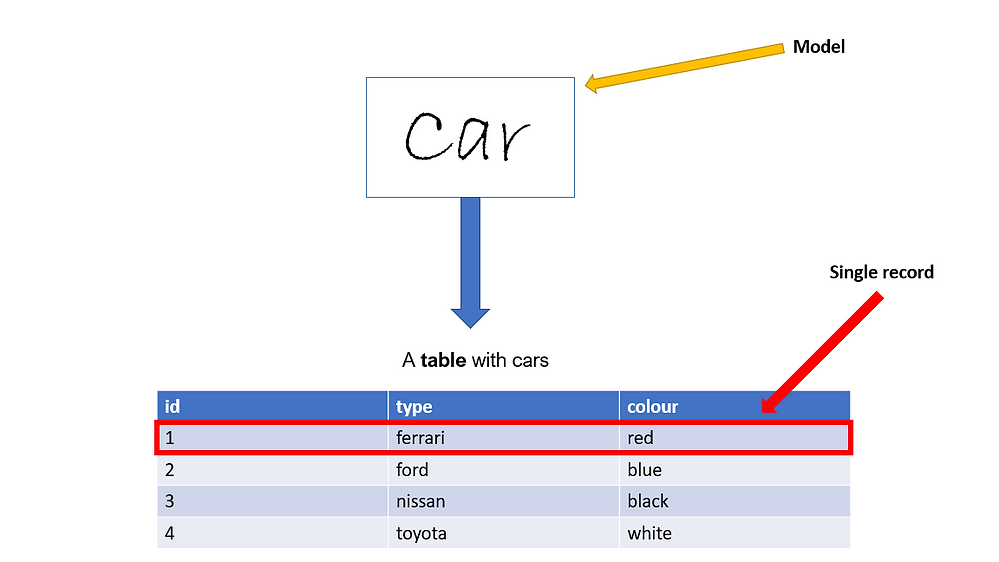
Now let's say that you don't want to read all the records from Car, but you would rather like to read a single record - perhaps, you want to get your first record that has data about a Ferrari that also happens to be red.
To do this you would need to use the get() function along with one of the car parameters. As you can see we are searching for a record whose id = 1... Which will get a record whose id is equal to 1.
Moreover, the get function will search all the rows in the table and return the first result.
The code below is how we would read a single record.
# views.py
car = Car.objects.objects.get(id=1)
DONE! - We are now able to read records from our table.
Python Django: Ultimate Beginners Course
Master Django development from scratch with theory and hands-on examples, building and deploying web applications.
🎓 Course available on Udemy now!