What are Django models?
A Django model is a built-in feature that is used to create tables and fields. These tables and fields are used to represent data that can be managed and accessed with Django. Simply put, models define the structure of the data that is stored.
Let's get started.
So, let's say that we want to keep track of data that is associated with different kinds of cars.
In this case, our model would be Car.
1) Creating a simple model in Django
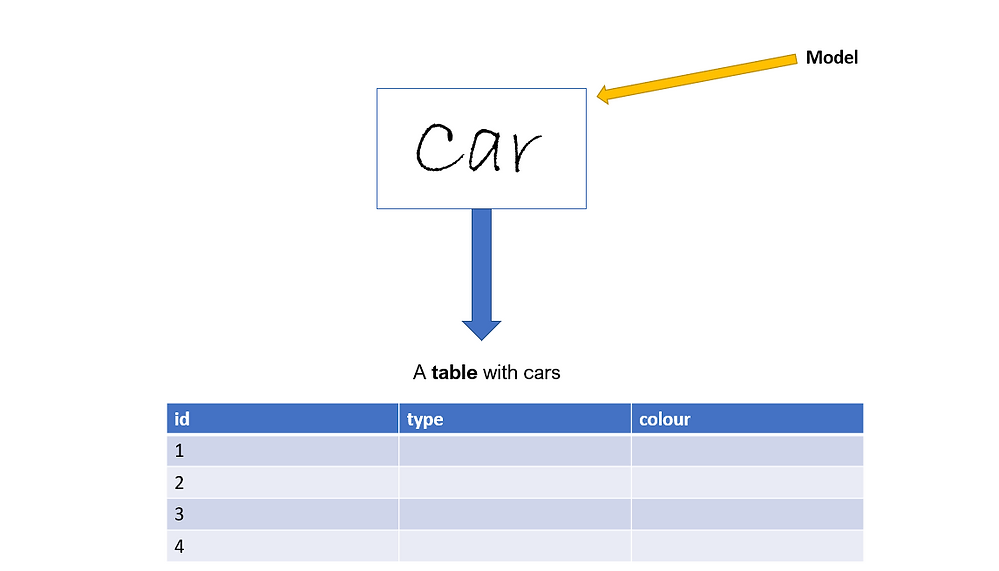
The code below shows how we would create our model Car and the table structure for it.
# - models.py
from django.db import models
class Car(models.Model):
car_type = models.CharField(max_length=30)
colour = models.CharField(max_length=30)
First of all, we navigate to our models.py file in Django and import models.
We then create our model, which is also known as a class. Since our model is Car, it would be appropriate to name it that.
We then create our fields, we have two of them, namely type and colour. The id field is generated by default when we create our model.
Next, it's important to decide in what form the data for our fields will be stored. Since we are just simply storing strings we can use CharField and set a max_length of 30 characters.
Once we have completed our model it is important that we migrate and push our changes.
To do so, we perform the following commands (1):
python manage.py makemigrations
python manage.py migrate
DONE! - Your model has been migrated and created!
2) Creating records for our table
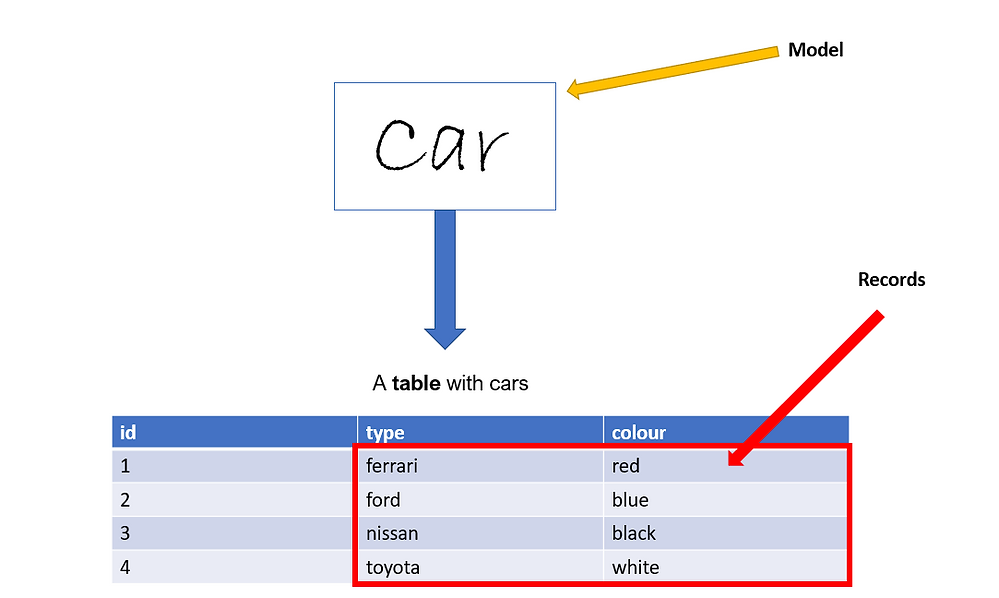
The next step involves adding records to our tables by invoking the create() function, which helps us to populate the table from above.
Car.objects.create(car_type='ferrari', colour='red')
Car.objects.create(car_type='ford', colour='blue')
Car.objects.create(car_type='nissan', colour='black')
Car.objects.create(car_type='toyota', colour='white')
DONE! - We have created records for our Model - Car